So, today I am going to demonstrate all the necessary steps required to accomplish this task (i.e. adding blog posts on homepage).
Step 1) You need "Source code" to perform these steps (NOT web version)
Step 2) We need to create a view
Go to: Nop.Web / View / Blog
Add a new view and name it: "HomePageBlog.cshtml"
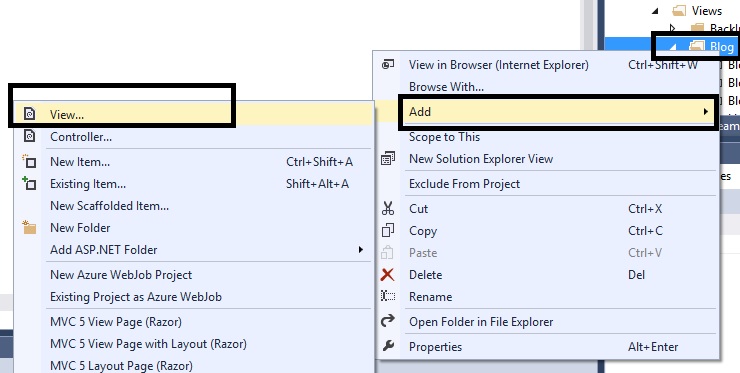
Step 3) Add this code in your HomePageBlog.chtml view page:
@
using
Nop.Web.Models.Blogs;
@
using
Nop.Web;
@
using
Nop.Web.Extensions;
<hr />
<center>
<h2>Recent Blog Posts</h2>
</center>
<hr />
<br/>
<h3>
@
foreach
(var item
in
Model.BlogPosts)
{
<a href=
"@Url.RouteUrl("
BlogPost
", new { blogPostId = item.Id, SeName = item.SeName })"
>
@item.Title
</a><br /><br />
}
</h3>
Step 4) Now, go to: Nop.Web / Controllers / BlogController.cs
Open the file "BlogController.cs"
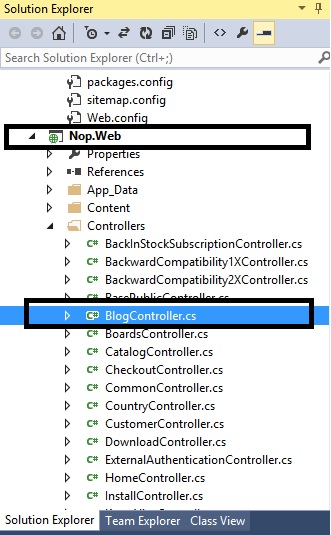
Step 5) We need to add a method "HomePageBlog"
public
ActionResult HomePageBlog(BlogPagingFilteringModel command)
{
var model =
new
BlogPostListModel();
model.WorkingLanguageId = _workContext.WorkingLanguage.Id;
if
(command.PageSize <= 0) command.PageSize = _blogSettings.PostsPageSize;
if
(command.PageNumber <= 0) command.PageNumber = 1;
DateTime? dateFrom = command.GetFromMonth();
DateTime? dateTo = command.GetToMonth();
IPagedList<BlogPost> blogPosts;
blogPosts = _blogService.GetAllBlogPosts(_storeContext.CurrentStore.Id,
_workContext.WorkingLanguage.Id,
dateFrom, dateTo, command.PageNumber - 1, 3);
model.PagingFilteringContext.LoadPagedList(blogPosts);
model.BlogPosts = blogPosts
.Select(x =>
{
var blogPostModel =
new
BlogPostModel();
PrepareBlogPostModel(blogPostModel, x,
false
);
return
blogPostModel;
})
.ToList();
return
PartialView(model);
}
Step 6) The number of blog posts you would like on your homepage can be controlled by this line above:
dateFrom, dateTo, command.PageNumber - 1, 3);
In this example, I am displaying "3" blog posts
Step 7) Now, go to: Nop.Web / View / Home / Index.cshtml
Open the "Index.cshtml"
Add this to your homepage (where you would like to display the blog posts):
@Html.Action("HomePageBlog", "Blog")
Like this:
@{
Layout =
"~/Views/Shared/_ColumnsThree.cshtml"
;
}
<div
class
=
"page home-page"
>
<div
class
=
"page-body"
>
@Html.Widget(
"home_page_top"
)
@Html.Action(
"TopicBlock"
,
"Topic"
,
new
{ systemName =
"HomePageText"
})
@Html.Action(
"HomepageCategories"
,
"Catalog"
)
@Html.Action(
"HomepageProducts"
,
"Product"
)
@Html.Action(
"HomepageBestSellers"
,
"Product"
)
@Html.Action(
"HomePageNews"
,
"News"
)
@Html.Action(
"HomePagePolls"
,
"Poll"
)
@Html.Widget(
"home_page_bottom"
)
@Html.Action(
"HomePageBlog"
,
"Blog"
)
</div>
</div>
Step 8) That's all! Build your project and you should see the blog posts on your homepage
(You can add the blog post to any page like this and add your custom design to it)
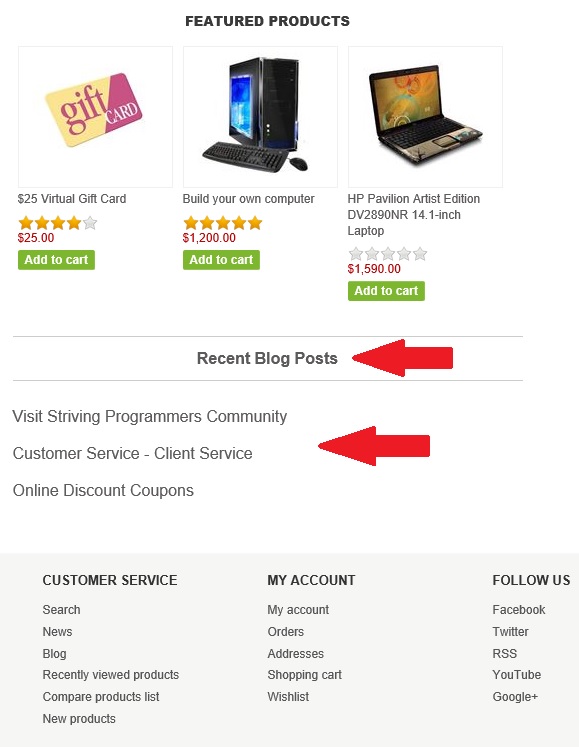
Version Information: I am using nopCommerce 3.5 version for this example.
Hope it helps!
My way was a bit different. I created a HomePageBlog model with a BlogPostModel list. From the controller I grabbed the latest 3 posts using _blogService, and looped through them passing the relevant parameters to my new model (Title, SeName, Date, BodyOverview <- which I believe is new to nop 3.6).
In my view I basically laid it out like the HomePageNewsItems are.
Thought I'd share - wish I found this before I went through all that work, but I also must say it was rewarding figuring it out!
I really like this information and i usually want to make new website with this blog functionality.